Enable/Disable Screenshare
Dyte allows you to enable multiple users to share their screens during a meeting session. To integrate screen sharing in your application, go through the following steps:
Usage​
Methods/Properties Core​
Once users have the permissions to share their screen, they need to be able to start or stop sharing their screens. Use the Core SDK to enable or disable screen sharing for the user. Follow the implementation based on the development platform you are using.
- Javascript
- React
- React Native
- Flutter
- Android
- iOS
// Enable Screenshare
await meeting.self.enableScreenShare();
// Disable Screenshare
await meeting.self.disableScreenShare();
You can also define defaults for screen sharing during the client initialisation. Check out the local user reference for more details.
const { meeting } = useDyteMeeting();
// Enable Screenshare
await meeting.self.enableScreenShare();
// Disable Screenshare
await meeting.self.disableScreenShare();
You can also define defaults for screen sharing during the client initialization. Check out the local user reference for more details.
const { meeting } = useDyteMeeting();
// Enable Screenshare
await meeting.self.enableScreenShare();
// Disable Screenshare
await meeting.self.disableScreenShare();
For iOS you need to complete a one time setup described here described here
// Enable Screenshare
dyteClient.localUser.enableScreenShare();
// Disable Screenshare
dyteClient.localUser.disableScreenShare();
// Get current status
dyteClient.localUser.screenShareEnabled;
// Enable Screenshare
meeting.localUser.enableScreenShare();
// Disable Screenshare
meeting.localUser.disableScreenShare();
// Get current status
meeting.localUser.screenShareEnabled;
After completing the screensharing setup described here
// Enable Screenshare
meeting.localUser.enableScreenShare();
// Disable Screenshare
meeting.localUser.disableScreenShare();
// Get current status
meeting.localUser.screenShareEnabled;
Events Core​
- Javascript
- React
- React Native
meeting.self.on(
'screenShareUpdate',
({ screenShareEnabled, screenShareTracks }) => {
if (screenShareEnabled) {
// handle screen share start
} else {
// handle stop screen share
}
}
);
const { meeting } = useDyteMeeting();
const screenshareEnabled = useDyteSelector((m) => m.self.screenShareEnabled);
// or using traditional event listeners
meeting.self.on(
'screenShareUpdate',
({ screenShareEnabled, screenShareTracks }) => {
if (screenShareEnabled) {
// handle screen share start
} else {
// handle stop screen share
}
}
);
const { meeting } = useDyteMeeting();
const screenshareEnabled = useDyteSelector((m) => m.self.screenShareEnabled);
// or using traditional event listeners
meeting.self.on(
'screenShareUpdate',
({ screenShareEnabled, screenShareTracks }) => {
if (screenShareEnabled) {
// handle screen share start
} else {
// handle stop screen share
}
}
);
Components UI Kit​
If you don't want to create your own button to toggle screen sharing, use the default button provided by our UI Kits.
- Web Components
- React
- Angular
- React Native
<dyte-screen-share-toggle size="sm" class="dyte-el"></dyte-screen-share-toggle>
<dyte-screen-share-toggle size="lg" class="dyte-el"></dyte-screen-share-toggle>
<dyte-screen-share-toggle
variant="horizontal"
class="dyte-el"
></dyte-screen-share-toggle>
<script>
const elements = document.getElementsByClassName('dyte-el');
for (const el of elements) {
el.meeting = meeting;
}
</script>
import { DyteScreenShareToggle } from '@dytesdk/react-ui-kit';
<Center>
<DyteScreenShareToggle size="sm" meeting={meeting} />
<DyteScreenShareToggle size="lg" meeting={meeting} />
<DyteScreenShareToggle variant="horizontal" size="sm" meeting={meeting} />
</Center>;
<dyte-screen-share-toggle size="sm" class="dyte-el"></dyte-screen-share-toggle>
<dyte-screen-share-toggle size="lg" class="dyte-el"></dyte-screen-share-toggle>
<dyte-screen-share-toggle
variant="horizontal"
class="dyte-el"
></dyte-screen-share-toggle>
import { DyteScreenShareToggle } from '@dytesdk/react-native-ui-kit';
<Center>
<DyteScreenShareToggle size="sm" meeting={meeting} />
<DyteScreenShareToggle size="lg" meeting={meeting} />
<DyteScreenShareToggle variant="horizontal" size="sm" meeting={meeting} />
</Center>;
Configuration​
Multiple Screenshare Preset​
Configure the number of people who can screenshare at once using preset configuration
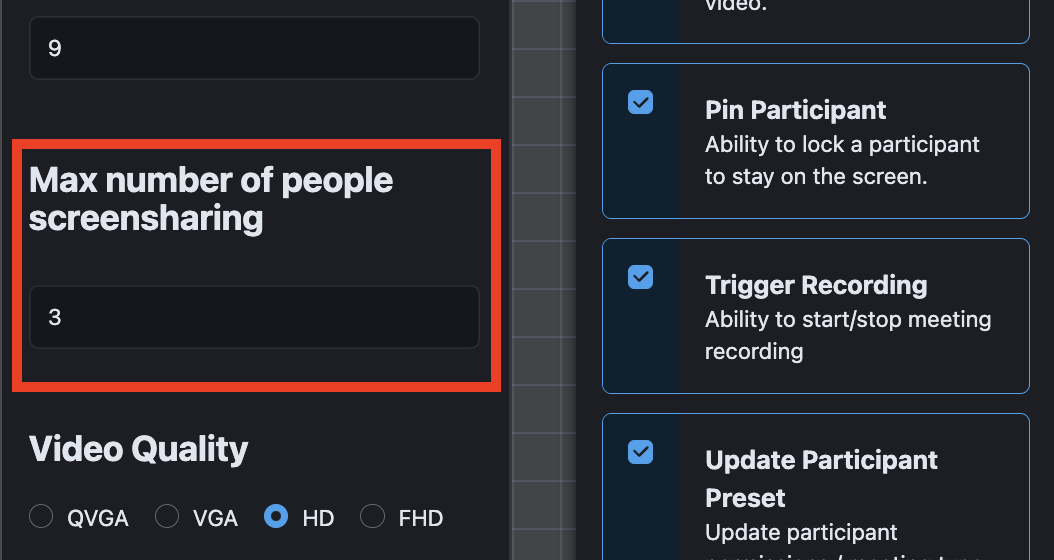
Preferred panel CoreWeb​
On web browsers, a user can choose between sharing a tab, a window or the entire screen. While there is no browser API to restrict to a specific surface type you can set a preferred display surface
- Javascript
- React
meeting = await DyteClient.init({
authToken,
defaults: {
screenShare: {
displaySurface: 'window' | 'monitor' | 'browser',
},
},
});
const [meeting, initMeeting] = useDyteClient();
const join = () => {
initMeeting({
authToken,
defaults: {
screenShare: {
displaySurface: 'window' | 'monitor' | 'browser',
},
},
});
};