Quickstart
This quickstart shows how to use Dyte's Flutter UI Kit SDK to add live video and audio to your Flutter applications.
For getting started quickly, you can use our sample code. You can clone and run a sample application from the Flutter UI Kit Sample App GitHub repository.
Objective
You’ll learn how to:
- Install the Dyte SDK
- Configuring Android & iOS permissions
- Initialize the SDK
- Configure a Dyte meeting
- Launch the meeting UI
Before Getting Started
Make sure you've read the Getting Started with Dyte topic and completed the steps in the Integrate Dyte section. You must complete the following steps:
- Create a Dyte Developer Account
- Create Presets
- Create a Dyte Meeting
- Add Participant to the meeting
Step 1: Install the SDK
Install the SDK from pub.dev.
flutter pub add dyte_uikit
Step 2: Configure permissions for Android and iOS
Perform the following steps:
Android
Set compileSdkVersion 33
and minSdkVersion 21
inside build.gradle
file at the <project root>/android/app/build.gradle
file.
defaultConfig {
...
compileSdkVersion 33
minSdkVersion 21
...
}
And change the kotlin version to 1.9.0
ext.kotlin_version = '1.9.0'
iOS
- Set minimum deployment target for your Flutter app to 13.0 or higher.
platform :ios, '13.0'
- Add the following keys to your
Info.plist
file, located in<project root>/ios/Runner/Info.plist
:
/* Attach the permission to use camera & microphone. */
<key>NSBluetoothPeripheralUsageDescription</key>
<string>We will use your Bluetooth to access your Bluetooth headphones.</string>
<key>NSBluetoothAlwaysUsageDescription</key>
<string>We will use your Bluetooth to access your Bluetooth headphones.</string>
<key>NSCameraUsageDescription</key>
<string>For people to see you during meetings, we need access to your camera.</string>
<key>NSMicrophoneUsageDescription</key>
<string>For people to hear you during meetings, we need access to your microphone.</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>For people to share, we need access to your photos.</string>
- In iOS, if you are allowing user to download attachments in chat, add the following permissions in your app's Info.plist:
<key>LSSupportsOpeningDocumentsInPlace</key>
<true/>
<key>UIFileSharingEnabled</key>
<true/>
Step 3: Configure a Dyte meeting
To initiate Dyte Meeting for any participant you just need to pass authToken
as an argument. You can get the authToken
via the Add Participant API.
After getting the authToken
, you need to create the DyteMeetingInfoV2
object as follows:
Name | Description |
---|---|
authToken | After you've created the meeting, add each participant to the meeting using the Add Participant API (The presetName created earlier must be passed in the body of the Add Participant API request) The API response contains the authToken . |
baseUrl | The base URL of the Dyte server. Default value is https://api.dyte.io/v2 . This is an optional argument. |
enableAudio | A boolean value to enable or disable audio in the meeting. Default value is true . This is an optional argument. |
enableVideo | A boolean value to enable or disable video in the meeting. Default value is true . This is an optional argument. |
final meetingInfo = DyteMeetingInfoV2(
authToken: '<authToken>',
baseUrl: 'https://api.dyte.io/v2', // optional argument, if you want to pass your own baseUrl
enableAudio: false, // optional argument, to enable or disable audio in the meeting
enableVideo: false, // optional argument, to enable or disable video in the meeting
);
Step 4: Initialize the SDK
The DyteUIKit
is the main class of the SDK. It is the entry point and the only
class required to initialize Dyte UI Kit SDK. To initialize it we have to pass DyteUIKitInfo
object as an argument.
/* Passing the DyteMeetingInfoV2 object `meetingInfo` you created in the Step 3,
*/
final uikitInfo = DyteUIKitInfo(
meetingInfo,
// Optional: Pass the DyteDesignTokens object to customize the UI
designToken: DyteDesignTokens(
colorToken: DyteColorToken(
brandColor: Colors.purple,
backgroundColor: Colors.black,
textOnBackground: Colors.white,
textOnBrand: Colors.white,
),
),
);
final uiKit = DyteUIKitBuilder.build(uiKitInfo: uikitInfo, context: context);
You can learn more about customization of the uikit in the Design System section.
Step 5: Launch the meeting UI
To launch the meeting you can simply use the object returned after calling build
method. The uikit
above is a widget itself.
You can push this widget as a page to start the flow of prebuilt Flutter UI Kit.
import 'package:dyte_uikit/dyte_uikit.dart';
import 'package:flutter/material.dart';
class DyteMeetingPage extends StatelessWidget {
const DyteMeetingPage({super.key});
Widget build(BuildContext context) {
...
// Push this widget as page in your app
// Earlier it was uikit.loadUI() which is now deprecated,just use uikit.
return uiKit;
}
}
Voila! You're all done. Here is the pictorial representation of all the configuration options passed.
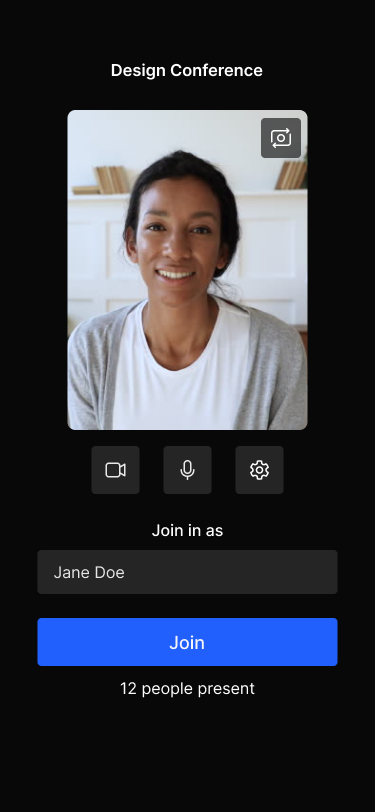
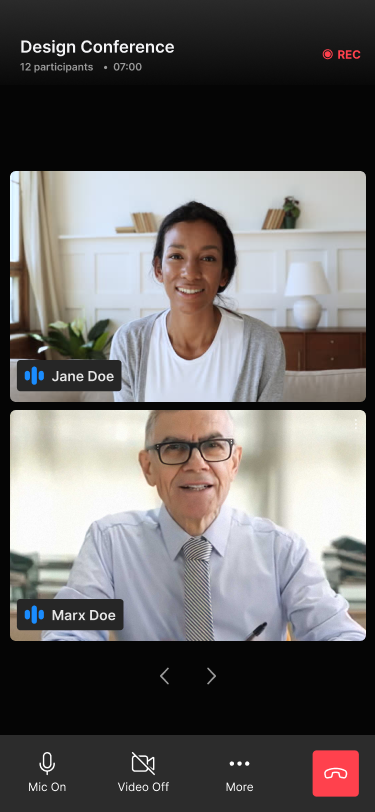
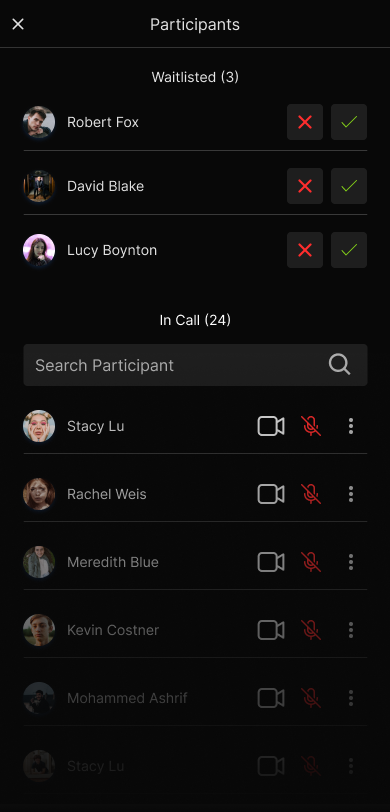
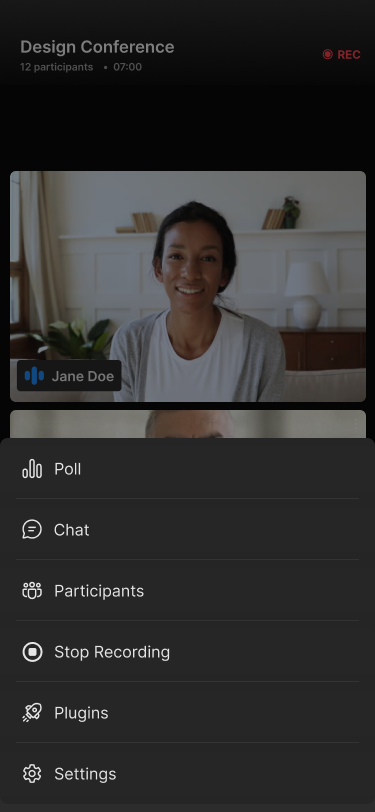
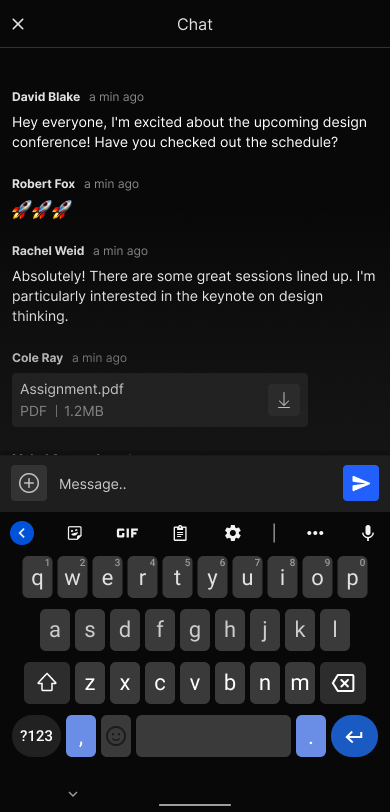
<head>
<title>Flutter Quickstart</title>
<meta name="description" content="Get started quickly with Dyte's Flutter integration. Follow our quickstart guide for seamless integration and development."/>
</head>