Edit user name
A common use case of pre-call UI is to give the user a option to set / edit their name.
Permissions
Requires meeting.self.permissions.canEditDisplayName
to be true
In the preset editor, ensure Miscellaneous > Edit Name
is toggled enabled.
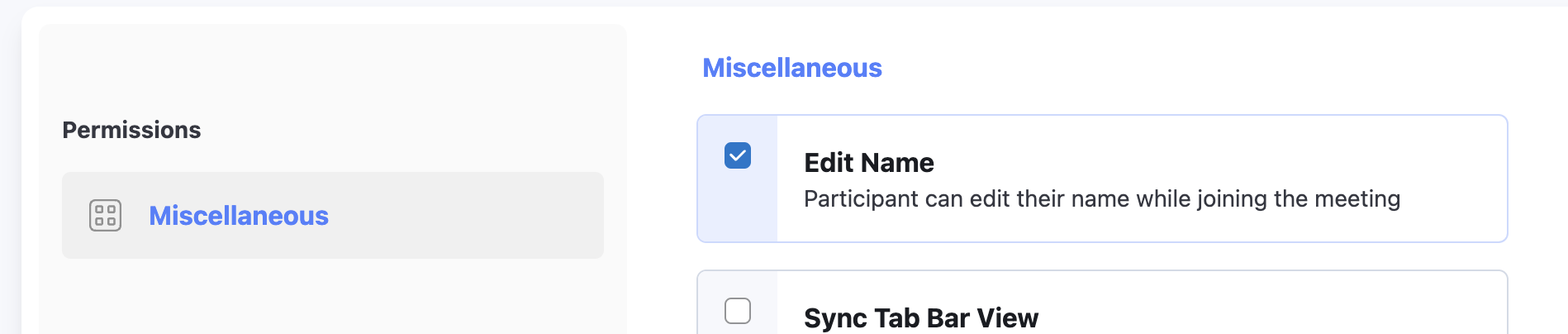
File: CustomMeetingPreview.tsx
We add a <input>
element for entering the participant name. We should not show this input if the user doese not have permission to edit name (permissions.canEditDisplayName
)
await meeting.self.setName(participantName);
sets the new name for the participant.
At the end, we let user join the meeting using await meeting.join();
.
LIVE EDITOR
import { useDyteMeeting, useDyteSelector } from "@dytesdk/react-web-core";
import { DyteButton } from "@dytesdk/react-ui-kit";
import { useState, useEffect } from "react";
export default function CustomMeetingPreview() {
const { meeting } = useDyteMeeting();
const permissions = useDyteSelector((m) => m.self.permissions);
const [participantName, setParticipantName] = useState("");
useEffect(() => {
if (!meeting) {
return;
}
setParticipantName(meeting.self.name);
}, [meeting]);
return (
<div
className="h-full w-full flex flex-col items-center justify-center"
style={{ minHeight: "400px" }}
>
<div className="flex w-full items-center justify-around p-[10%]">
<div></div>
<div className="flex w-1/4 flex-col justify-between">
<div className="flex flex-col items-center">
<p>Joining as</p>
</div>
{permissions.canEditDisplayName && (
<input
placeholder="Your name"
className="mb-10 rounded-sm border p-2.5 focus:border-blue-500"
value={participantName}
onChange={(event) => setParticipantName(event.target.value)}
/>
)}
<DyteButton
kind="wide"
size="lg"
style={{ cursor: participantName ? "pointer" : "not-allowed" }}
onClick={async () => {
if (participantName) {
if (permissions.canEditDisplayName) {
await meeting.self.setName(participantName);
}
await meeting.join();
}
}}
>
Join
</DyteButton>
</div>
</div>
</div>
);
}